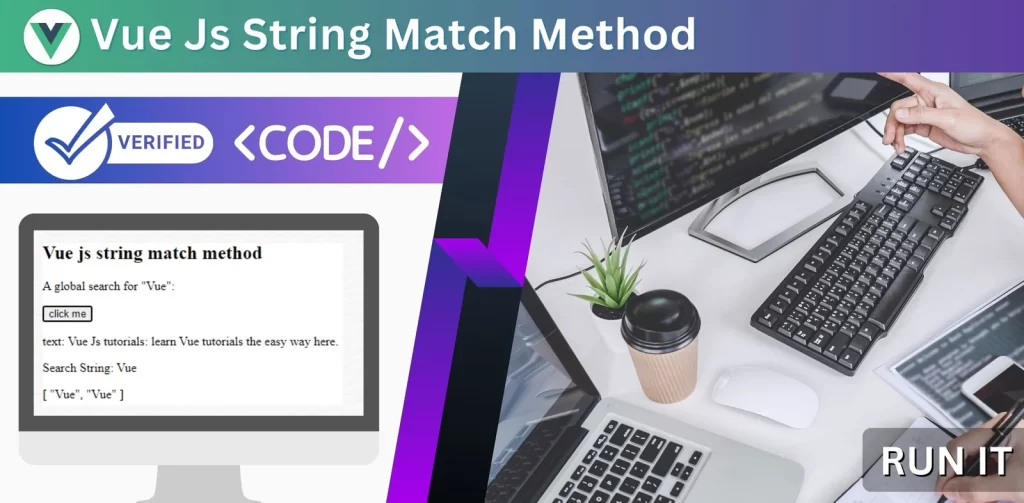
Vue Js String Match Method: Vue JS String match method is used to search a string for a specified pattern and return an array of results When using the match() method, it is important to note that if no matching string pattern is found in the searched string, it will return a null value With an example, we will learn how to use the string match function with Vue JS in this tutorial.
How do I search a string in Vue JS?
The match() method in Vue Js can be used to search for a string within a given text.
Vue Js Search String Example
<div id="app">
<button @click="myFunction">click me</button>
<p>text: {{text}}</p>
<p>Search String: Vue</p>
<p>{{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
text : 'Vue Js tutorials: learn Vue tutorials the easy way here. ',
results:''
}
},
methods:{
myFunction(){
this.results = this.text.match(/Vue/g);
},
}
}).mount('#app')
</script>
Output of above example
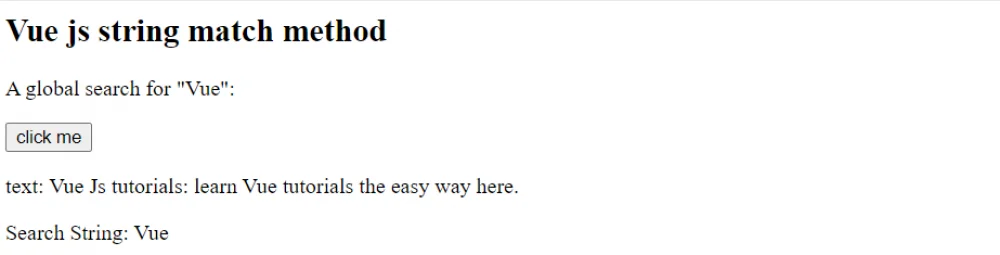
Exact Substring Within a String: How to Use Vue.js for Global, Case-Insensitive Search
String.match(/substring/gi) in Vue.js allows you to quickly search for a case-insensitive substring, as shown below.
In Vue Js, determine whether a string contains a specific word.
<div id="app">
<button @click="myFunction">click me</button>
<p>text: {{text}}</p>
<p>Search String: Vue</p>
<p>{{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
text : 'Vue Js tutorials: learn vue tutorials the easy way here. ',
results:''
}
},
methods:{
myFunction(){
this.results = this.text.match(/Vue/gi);
},
}
}).mount('#app')
</script>
Output of above example
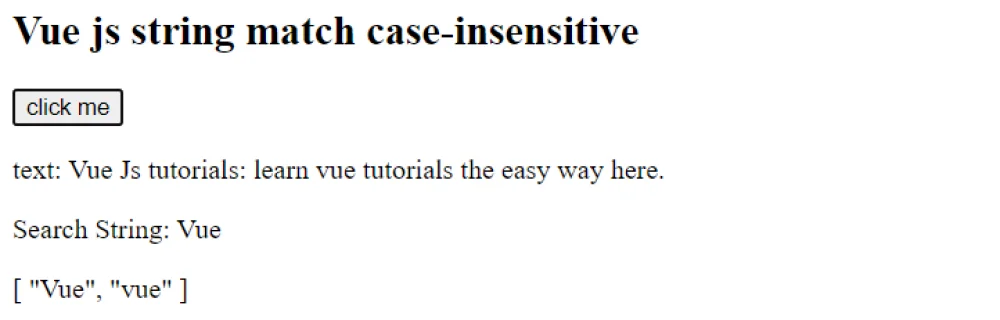